In this blog post, we will learn how to invoke a flow from lightning web components through lightning-flow. And will show you how to fire toast message in flow using lwc and navigate to the record detail page in flow.
Lightning Web Components (LWCs) are a powerful way to build custom Salesforce applications. They allow you to create reusable, declarative components that can be used in any Salesforce page or record.
Table of Contents
Use Case
Once the contact record is created from the screen flow we will fire a toast message and navigate to the contact to record detail page.
Read the best salesforce Books: Click Here.
How to Fire a Toast Message in Flow?
Will start first with creating a screen flow, where the user enters some inputs to create a contact.
Go to Flows and choose Screen Flow. To create a contact I’m choosing Name, Email, and Description as input parameters from users.
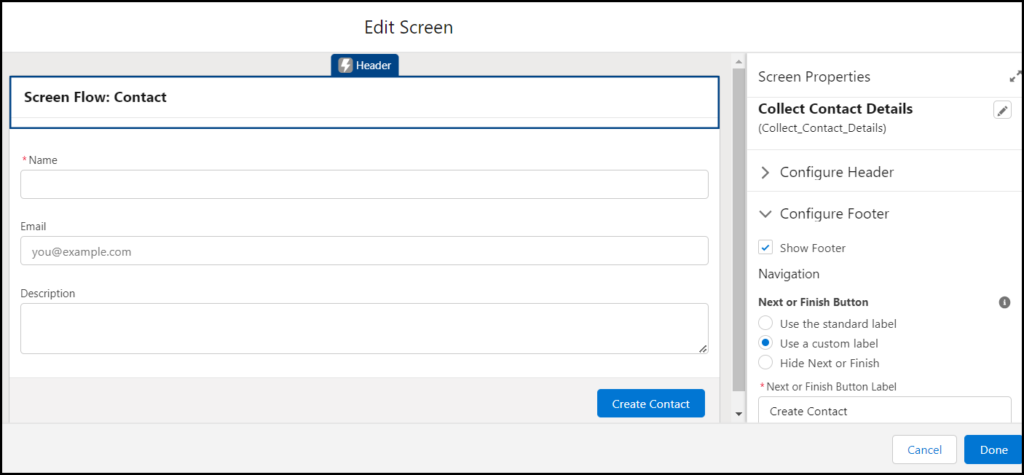
Next will choose the create record element to create a contact record. Before that will create a variable in flow to store the created contact ID and this record ID will be used to navigate to the record page using lwc.
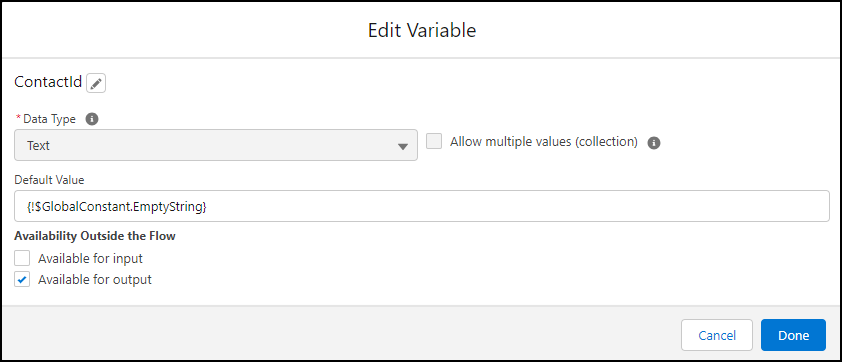
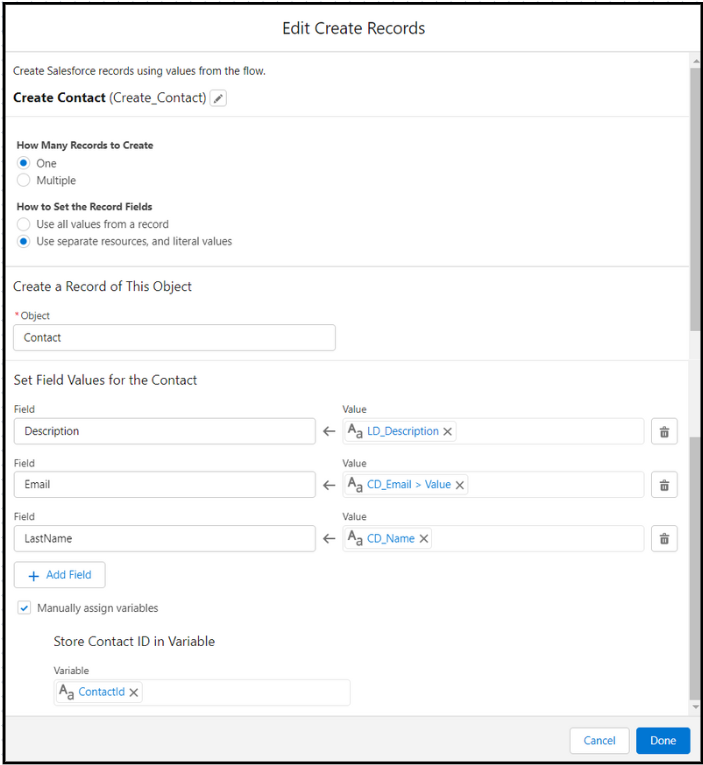
Whoo!! We are done with our screen flow and it looks like below.
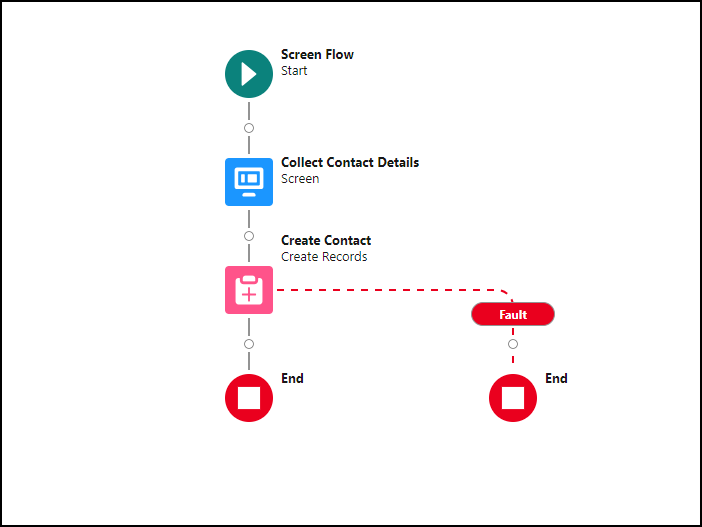
Now we are done with Flow. So let’s create a Lightning wed component to invoke this flow.
To invoke a flow from an LWC, you can use the lightning-flow
. The lightning-flow
component has the following attributes:
flow-api-name
: This is the API name of the flow that you want to invoke.flow-input-variables
: This is an array of objects that represent the input variables for the flow. Each object must have aname
property and avalue
property.onstatuschange
: This is a callback function that is called when the status of the flow changes.
Usage Considerations
The lightning-flow
component only supports active flows for the flowApiName
attribute.
If you have custom Lightning Web Components or Aura components in your flow, then you won’t be able to use lightning-flow
on Experience Cloud sites that use Lightning Web Runtime.
Check what all the changes parameters are available. Let’s create an LWC component.
contactToastMsgLWC.Html
<template>
<lightning-flow flow-api-name='Screen_Flow_Contact' onstatuschange={handleStatusChange} >
</lightning-flow>
</template>
contactToastMsgLWC.Js
import { LightningElement} from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { NavigationMixin } from 'lightning/navigation';
export default class ContactToastMsgLWC extends NavigationMixin(LightningElement){
handleStatusChange(event) {
console.log("event detail",event.detail.status);
if(event.detail.status === "FINISHED") {
//Get the flow output variable and store it.
const outputVariables = event.detail.outputVariables;
for(let i= 0; i < outputVariables.length; i++) {
const outputVar = outputVariables[i];
//contactId is a variable created in flow.
if(outputVar.name === 'ContactId') {
console.log(outputVar.value);
if(outputVar.value != null){
//Call ShowToast Function
this.showToast("Success","Contact Created Sucessfully","success");
//Pass the contactId variable value to navigateToRecord.
this.navigateToRecord(outputVar.value);
}else{
console.log('contact is not created');
}
}
}
}
if(event.detail.status === "ERROR") {
this.showToast("error","Error occurred while creation of contact","error");
}
}
//Toast Message
showToast(title,message,variant) {
const evt = new ShowToastEvent({
title,
message,
variant
});
this.dispatchEvent(evt)
}
//Navigate to contact detail page.
navigateToRecord(recordId) {
this[NavigationMixin.Navigate]({
type: 'standard__recordPage',
attributes: {
recordId,
actionName: 'view'
}
});
}
}
contactToastMsgLWC.Js-meta.xml
<?xml version="1.0"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>57.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__FlowScreen</target>
<target>lightning__RecordPage</target>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Outcome
Add the LWC component to any page in Salesforce. Currently, I have added it to the record page.
Related Quick Links
- Read the best salesforce Books: Click Here.
- HTTP Callout in Salesforce Flow Without Code [GET & POST]
- How to Invoke a Flow from a Lightning Web Component
- How to update child records using salesforce flow
- How to Assign records to Queue using Salesforce Flow?
- How to create a Data Table in Salesforce Flow : Complete Guide.
- How to retrieve record Type Id in flow
- How to navigate record detail page in flow
- How to send email using flow in salesforce
- How to Display Icon In Lightning Datatable
- How to Create Record in lightning Component
- How to Delete Multiple Records Using Checkbox In Lightning Component.
9 thoughts on “How to Invoke a Flow from a Lightning Web Component”